How to VB.Net Arrays
An array is a data structure that allows you to store and manipulate a fixed-size collection of elements of the same type. Arrays provide a convenient way to work with multiple values of the same data type under a single variable name.
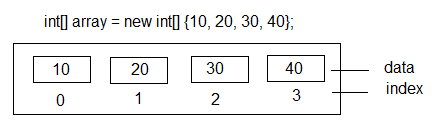
The commonly using functions are follows :
Declaring an Array
To declare an array in VB.NET, you need to specify the data type of the elements and the size of the array. Here's an example of declaring an array of integers:
In this example, we declare an array called "numbers" that can hold 5 integer values (indexed from 0 to 4).
Initializing an Array
After declaring an array, you can initialize it with values using various methods.
Inline initialization:Accessing Array Elements
Array elements can be accessed using their index, which starts from 0 and goes up to the length of the array minus 1.
Modifying Array Elements
You can modify the values of array elements by assigning new values to them using their index.
Iterating through an Array
You can loop through the elements of an array using a For loop or a For Each loop. Here's an example using a For Each loop:
Array Properties and Methods
Arrays in VB.NET provide various properties and methods to work with. Here are a few commonly used ones:
- Length Property: Returns the number of elements in the array.
- Rank Property: Returns the number of dimensions in the array.
- GetUpperBound Method: Returns the highest index value for a particular dimension.
Multidimensional Arrays
VB.NET also supports multidimensional arrays, where you can have more than one dimension.
In this example, we declare a 2-dimensional array called "matrix" with 3 rows and 3 columns.
How to check if a value exists in an array ?
The provided VB.NET source code showcases a selection of frequently utilized functions.
Full Source VB.NETConclusion
Arrays in VB.NET provide a powerful way to store and manipulate collections of values. They are widely used in various programming scenarios to store data efficiently and perform operations on multiple values simultaneously.