How to send email from VB.NET
What is SMTP ?
SMTP (Simple Mail Transfer Protocol) is a widely adopted protocol designed for the purpose of sending electronic mail (email) messages between servers. As an integral part of the Internet's infrastructure, SMTP has established itself as the de facto standard for transmitting email across different systems.
SMTP operates on the principle of reliable and efficient mail delivery, ensuring that email messages are successfully transferred from the sender's email server to the recipient's email server. This process involves a series of well-defined steps and commands, allowing for seamless communication between the involved servers.
By default, SMTP utilizes TCP (Transmission Control Protocol) as the underlying transport protocol. It operates on TCP port 25, which serves as the standard port for SMTP communication. However, it's important to note that some Internet Service Providers (ISPs) may implement different port assignments for SMTP. In addition, there is a secure version of SMTP called SMTPS, which uses SSL (Secure Sockets Layer) or its successor, TLS (Transport Layer Security), to encrypt the communication between the email client and the email server. SMTPS typically operates on port 465, ensuring secure transmission of email data.
SMTP Servers
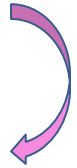
SMTP, an acronym for Simple Mail Transfer Protocol, offers a comprehensive suite of protocols designed to streamline the exchange of email messages between email servers. To establish connections with SMTP servers, they are typically identified by names conforming to the structure "smtp.domain.com" or "mail.domain.com." For instance, a Gmail account will reference its SMTP server as smtp.gmail.com.
The ubiquitous nature of SMTP is evident in its widespread adoption across diverse email systems that communicate over the Internet. This protocol serves as the backbone for transmitting messages from one server to another, ensuring reliable and efficient delivery. Once the messages have reached the recipient server, they can be accessed by email clients using either the POP (Post Office Protocol) or IMAP (Internet Message Access Protocol) protocols.
SMTP provides a vital link in the email communication chain, simplifying the intricate process of sending and receiving messages across networks. By adhering to SMTP's established guidelines, email servers can seamlessly communicate and relay messages, developing efficient and secure email transmission.
SMTP Auhentication
SMTP Authentication, commonly known as SMTP AUTH, represents a valuable extension to the SMTP (Simple Mail Transfer Protocol) framework. This extension empowers an SMTP client to authenticate itself by using a chosen authentication mechanism supported by the SMTP servers.
With the incorporation of SMTP AUTH, the client gains the ability to securely log in and establish its identity during the email transmission process. This authentication mechanism ensures that only authorized users can access and utilize the SMTP servers, bolstering the overall security and integrity of email communication.
By implementing SMTP AUTH, email clients can select from a range of supported authentication mechanisms, utilizing methods such as username and password combinations or other secure credentials. This flexibility ensures compatibility with various SMTP servers and enables a seamless integration between the client and server, developing reliable and secure email transmission.
The inclusion of SMTP Authentication serves as a crucial enhancement to the SMTP protocol, fortifying the authentication process and elevating the overall level of trust and security in email communication.
How do I send mail using VB.Net?
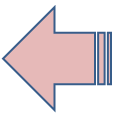
The SMTP protocol is utilized for sending email messages. SMTP, an acronym for Simple Mail Transfer Protocol, serves as the underlying framework responsible for the reliable transmission of emails. To facilitate email sending in VB.NET, the System.Net.Mail namespace is employed.
By instantiating the SmtpClient class and configuring its properties, such as Host and Port, VB.NET developers can establish a connection with the designated SMTP server. Although the default port for SMTP communication is typically 25, it is important to note that different mail servers may employ varying port configurations.
Send Email using Gmail in VB.Net
The Gmail SMTP server name is smtp.gmail.com and the port using send mail is 587 . Here using NetworkCredential for password based authentication.
Full Source VB.NETConclusion
You have to provide the informations like your gmail username and password , and the to address also.
- VB.NET Send email using CDOSYS
- How to find IP Address of Host
- How to read a URL Content
- VB.NET Socket Programming
- VB.NET Server Socket Program
- VB.NET Client Socket Program
- VB.NET MultiThreaded Socket Programming
- VB.NET MultiThreaded Server Socket Programming
- VB.NET MultiThreaded Client Socket Programming
- VB.NET Chat Server Program
- VB.NET Chat Server
- VB.NET Chat Client
- VB.NET Email Attachment
- How to VB.Net Web Browser