Delete from datagridview by Right click
There are numerous approaches available to effectively execute a right-click action for selecting a specific row within a Datagridview and subsequently displaying a contextual menu to facilitate its deletion. In this particular instance, we are employing the Datagridview CellMouseUp event to successfully identify the desired row, and using the contextMenuStrip1_Click event within the vb.net programming language to seamlessly delete the selected row from the Datagridview.
CellMouseUp event
By utilizing the CellMouseUp event in the Datagridview, we can effortlessly capture the user's right-click action, enabling us to precisely detect the targeted row. This event serves as a fundamental mechanism that empowers us to accurately identify the row on which the user intends to perform an action.
contextMenuStrip1_Click event
Following the row selection process, the contextMenuStrip1_Click event takes center stage. This event, which is linked to the corresponding contextMenuStrip component, serves as the trigger point for initiating the deletion operation. Once the user interacts with the contextual menu by clicking the appropriate delete option, the associated event is invoked, effectively eliminating the selected row from the Datagridview.
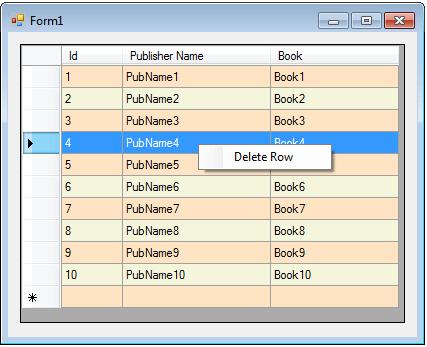
Select row in a dataGridView by Right click
First you should drag a contextMenuStrip from your toolbox to your form. Then you create a contextMenuStrip item "Delete Row".
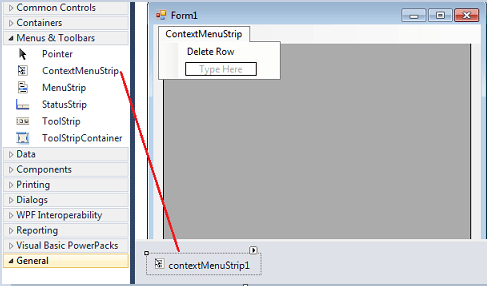
When the right mouse button is pressed, the initial step entails locating the index of the selected row, followed by the subsequent display of the contextMenuStrip. This process is facilitated through the implementation of the CellMouseUp event within the dataGridView component. By utilizing this event, the row index can be accurately identified, enabling the appropriate menu item to be shown.
To achieve this, a global variable called "rowIndex" is employed. This variable serves the purpose of storing the value of the row index, which will be utilized later on to delete the corresponding row. By assigning the value of the row index to this global variable within the CellMouseUp event, it becomes readily accessible for deletion operations at a later stage.
We can delete the selected row in the contextMenuStrip1_Click event by using the global rowIdex value.

- VB.NET DataGridView binding - Sql Server
- DataGridView binding - OLEDB in VB.NET
- DataGridView Sorting/Filtering in VB.NET
- DataGridView adding rows and columns in VB.NET
- DataGridView hiding rows and columns in VB.NET
- DataGridView ReadOnly rows and columns in VB.NET
- Adding Button to DataGridView in VB.NET
- Adding CheckBox to DataGridView in VB.NET
- Adding ComboBox to DataGridView in VB.NET
- Adding Image to DataGridView in VB.NET
- Adding ViewLink to DataGridView in VB.NET
- How to Paging in DataGridView
- How to Formatting in DataGridView
- How to DataGridView Template
- How to DataGridView Printing in VB.Net
- How to Export datagridview to Excel
- How to Import data from Excel to DataGridView
- Database operations in DatagridView
- DataGridView Autocomplete TextBox in VB.Net