DataGridView Autocomplete TextBox
Presenting data in a tabular format is a frequent requirement that you are likely to encounter in various programming tasks. In the field of VB.Net, the DataGridView control serves as a versatile solution for accomplishing this objective. This control offers a plethora of customization options, empowering you to tailor its appearance and functionality according to your specific needs, all through the utilization of its properties, methods, and events.
The DataGridView control within VB.Net boasts a rich set of configurable attributes that enable you to achieve a customized display. Through its properties, you can effortlessly manipulate various aspects of the control, such as the font, color, alignment, and size of the data cells, column headers, and row headers. Furthermore, you have the ability to control the visibility and ordering of columns, as well as the sizing and resizing behavior of both rows and columns. This level of configurability ensures that you can present your data in a visually appealing and user-friendly manner.
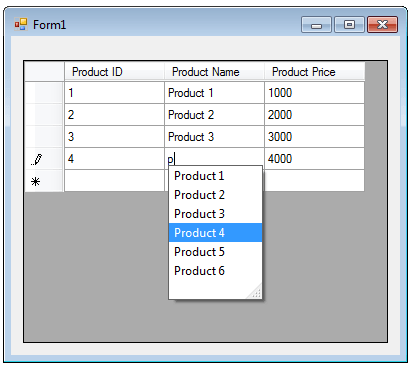
VB.Net DataGridView
In VB.Net you can display DataGridView in Bound mode, unbound mode and Virtual mode . Bound mode is suitable for managing data using automatic interaction with the data source. The data can be displayed in the DatagridView using TextBox, ComboBox, ListBox etc. While you display the data on TextBox, you can make your TextBoxes as Autocomplete TextBox in VB.Net.
AutoComplete in DataGridView
The TextBox control provides several properties, namely AutoCompleteCustomSource, AutoCompleteMode, and AutoCompleteSource, which facilitate the automatic completion of user input strings by comparing the entered prefix letters with the prefixes of strings stored in a data source.
AutoCompleteCustomSource property
The AutoCompleteCustomSource property allows you to specify a custom collection of strings that will be used for the autocomplete functionality. You can populate this collection with values from a data store, such as a database, an array, or any other data structure. By setting this property, the TextBox control will compare the prefix of the user's input with the prefixes of the strings in the specified collection to provide suggestions for autocompletion.
AutoCompleteMode property
The AutoCompleteMode property determines the mode of autocomplete behavior for the TextBox control. It offers different options, such as None, Suggest, Append, and SuggestAppend. When set to the Suggest mode, the TextBox will display a dropdown list of suggestions based on the entered prefix. In the Append mode, the TextBox will automatically append the remaining characters of the first suggestion to the user's input. The SuggestAppend mode combines both suggestions and appending behavior.
The AutoCompleteSource property is used to specify the source of the autocomplete suggestions. It can be set to values like CustomSource, FileSystem, FileSystemDirectories, AllUrl, HistoryList, RecentlyUsedList, or AllSystemSources. By selecting the appropriate source, you can define the origin of the suggestions that will be compared with the user's input.
The following VB.Net program shows how to create an AutoComplete Textbox inside a DataGridView.
Full Source VB.NET- VB.NET DataGridView binding - Sql Server
- DataGridView binding - OLEDB in VB.NET
- DataGridView Sorting/Filtering in VB.NET
- DataGridView adding rows and columns in VB.NET
- DataGridView hiding rows and columns in VB.NET
- DataGridView ReadOnly rows and columns in VB.NET
- Adding Button to DataGridView in VB.NET
- Adding CheckBox to DataGridView in VB.NET
- Adding ComboBox to DataGridView in VB.NET
- Adding Image to DataGridView in VB.NET
- Adding ViewLink to DataGridView in VB.NET
- How to Paging in DataGridView
- How to Formatting in DataGridView
- How to DataGridView Template
- How to DataGridView Printing in VB.Net
- How to Export datagridview to Excel
- How to Import data from Excel to DataGridView
- Database operations in DatagridView
- Delete row from datagridview by Right click