How to populate a combobox from Database | VB.Net
The DataSet serves as a comprehensive collection of DataTable objects, offering a robust framework for managing and interconnecting data through the use of DataRelation objects. In the context of VB.Net, the SqlDataAdapter object plays a key role by providing a convenient means of populating Data Tables within the DataSet. This powerful combination empowers developers to efficiently handle and manipulate data within their applications.
Moreover, the benefits of the DataSet extend beyond its role as a data container. It seamlessly integrates with various user interface elements, showcasing its versatility. For instance, developers can effortlessly populate a combo box by using the values stored within a DataSet. This functionality simplifies the process of populating the combo box, enhancing the overall user experience and reducing the complexity of data management tasks.
Bind data source to ComboBox
By utilizing the capabilities of the DataSet and the SqlDataAdapter, developers can create sophisticated data-driven applications with ease. The DataSet's ability to store and manage multiple tables, along with its support for data relations, empowers developers to build robust and interconnected data structures. Coupled with the convenience of populating combo boxes and other UI elements, the DataSet proves to be a valuable asset in the development of efficient and user-friendly applications.
Select Item from ComboBox
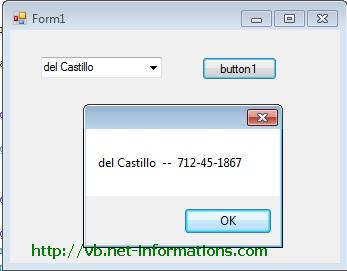
The following VB.Net program fetch the values from database and store it in a dataset and later bind to a combobox.
Binding a ComboBox to an Enum in VB.Net
Enums offer a powerful and efficient approach to managing collections of interconnected constants, allowing for easy organization and maintenance of code. With Enums, you can group related constants together, providing a clear and concise representation of their purpose.
One notable benefit of Enums is their seamless integration with user interface elements, such as ComboBoxes. By binding the Enum values to a ComboBox, you enable users to select options directly from a predefined list of Enum strings. This eliminates the need for manual input and reduces the likelihood of errors, as users can choose from a set of predefined and meaningful options.
The combination of Enums and ComboBoxes not only improves the readability and structure of your code but also enhances the user experience of your application. Users can effortlessly interact with ComboBoxes populated with Enum values, resulting in a more intuitive and user-friendly interface. This integration provides a streamlined and efficient means of interacting with your application, increasing user satisfaction and productivity.
Data Binding an Enum with Descriptions
The follwoing VB.Net program bind a combobox with Enum values.
Bind a ComboBox to a generic Dictionary in VB.Net
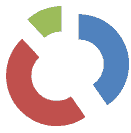
The Dictionary class provides a versatile and efficient solution for managing key-value pairs in a collection. With this data structure, each key serves as a unique identifier for its associated value, ensuring that there are no duplicate keys within the Dictionary. This uniqueness allows for quick and direct access to values based on their corresponding keys.
The Dictionary offers a wide range of functionalities for working with key-value pairs. You can easily add, remove, and update entries in the Dictionary, allowing for dynamic data manipulation. Retrieving values based on their keys is also highly optimized, resulting in fast and efficient lookup operations.
It's worth noting that while each key is unique, multiple keys can be associated with the same value. This flexibility enables various relationships and mappings between different data elements. For example, you can have a Dictionary where each key represents a person's name, and the associated value is their age. In this scenario, multiple people can have the same age, but each name would serve as a unique key.
The Dictionary class offers a wide range of methods and properties to efficiently work with key-value pairs. Whether you need to search for specific keys, iterate over the entries, or perform bulk operations, the Dictionary provides a comprehensive set of tools to handle various scenarios.
Overall, the Dictionary class is a powerful data structure that simplifies the management and organization of key-value pairs. Its efficient lookup operations and flexibility in handling relationships make it an essential tool for many programming tasks.
Dictionary as a Combobox Datasource
The following VB.Net program populating a Combobox from a Dictionary .
Full Source VB.NETChoosing the Right Method
- If you need to bind the combo box to the dataset for two-way data synchronization, or if you want to leverage data binding features, use the first method (DataSource and DataMember).
- If you need more control over individual items or don't need data binding functionalities, the second method (looping) might be preferable.
Additional Considerations
- You can also set the SelectedIndex property to choose the initially selected item in the combo box.
- Remember to handle errors while accessing the dataset or table.
Conclusion
By using the Dictionary class, you can conveniently store and retrieve data using key-value pairs, providing a reliable and efficient solution for various programming scenarios. The flexibility offered by this data structure allows for diverse applications, ranging from simple lookup operations to more complex data management tasks.
- What is ADO.NET Dataset
- How to Dataset with Sql Server
- How to Dataset with OLEDB Data Source
- Search Tables in a Dataset Sql Server
- Search Tables in a Dataset OLEDB Data Source
- Dataset table row count in SQL Server
- Dataset table row count - OLEDB Data Source
- How to find column definition - Sql Server
- How to find column definition - OLEDB Data Source
- How to create DataSet without Databse
- How to multiple tables in Dataset - Sql Server
- How to multiple tables in Dataset - OLEDB Data Source
- How to add relations between tables in a Dataset
- How to merge tables in a Dataset - Sql Server
- How to merge tables in a Dataset - OLEDB Data Source
- How many tables exist in a database - VB.NET