How to create Excel file in VB.Net
Automation to Excel empowers users to execute a variety of tasks, including the creation of new workbooks, data population, and chart generation. By utilizing COM interop, developers can use the power of Excel programmatically in VB.Net.
COM interop
The code example demonstrates the use of COM interop to establish a connection with Excel and automate the process of creating a new workbook. This involves using the appropriate classes, properties, and methods provided by the Excel object model to create and manipulate the workbook.
Excel interop assemblies
It's recommended to ensure that the necessary references and imports are included in the VB.Net project to access the Excel interop assemblies. This allows for seamless integration with Excel and access to its functionalities.
By following the code example and having Excel installed on your system, you can programmatically create Excel files, customize their content, and use the full capabilities of Excel within your VB.Net application.
Excel Library
In order to access the object model from Visual VB.NET, you have to add the Microsoft Excel 12.0 Object Library to your current project.
Create a new project and add a Command Button to your VB.Net Form.
How to use COM Interop to Create an Excel Spreadsheet
Form the following images you can find how to add Excel reference library in your VB.Net project.
Select reference dialogue from Project menu.
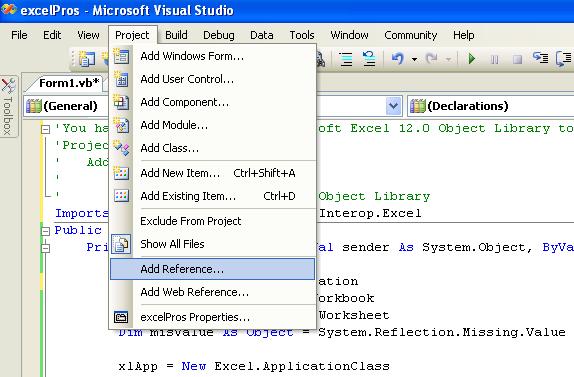
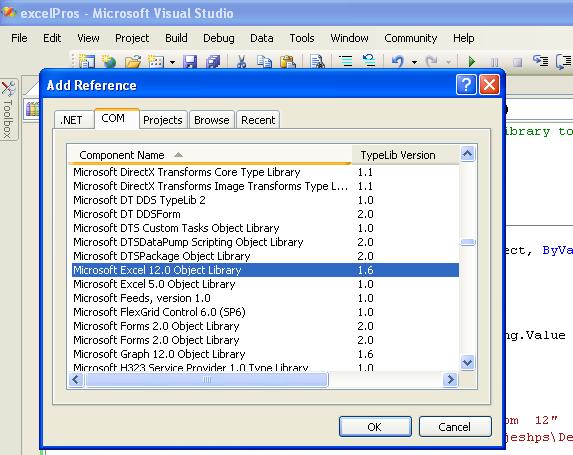
How to create an Excel Document Programmatically
First we have to initialize the Excel application Object in your VB.Net application.
Before creating new Excel Workbook, you should check whether Excel is installed in your system.
Then create new Workbook
After creating the new Workbook, next step is to write content to worksheet
In the above code we write the data in the Sheet1, If you want to write data in sheet 2 then you should code like this.
Save Excel file (SaveAs() method)t
After write the content to the cell, next step is to save the excel file in your system.
How to properly clean up Excel interop objects
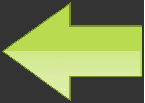
Finally, we have to properly clean up Excel interop objects or release Excel COM objects. Here we write a function to clean up the Excel Application, Excel Workbook and Excel Worksheet Objects.
Creating an Excel Spreadsheet Programmatically
Copy and paste the following source code in your VB.Net project file.
Full Source VB.NETConclusion
Through automation and COM interop, developers can utilize the capabilities of Excel within VB.Net to create, manipulate, and enhance Excel files programmatically. However, it's essential to have Excel installed on the system and properly handle any potential exceptions for the code to run successfully.
- How to open or read an existing Excel file in VB.NET
- How to read an Excel file using VB.Net
- Add new worksheet in Excel file - VB.Net
- Delete worksheet from an excel file - VB.Net
- How to Format Excel Page in VB.NET
- How to insert a Picture in Excel through programing on VB.NET
- How to insert a background Picture in Excel through VB.NET
- How to create a Chart in Excel in VB.NET
- How to export a Chart in Excel as Picture file from VB.NET
- How to Excel Chart in VB.NET Picture Box
- How to Excel DataBar in VB.NET
- How to Excel Data Validation Input Message
- Read and Import Excel File into DataSet or DataTable in vb.net
- How to insert cell data in an Excel file using OLEDB
- How to update cell data in an Excel file using OLEDB
- How to export from database to excel
- How to export from DataGridView to excel in VB.Net