Open and Edit Cells in an Excel file in VB.NET
To open and edit an Excel worksheet through VB.NET, you need to add the Microsoft Excel 12.0 Object Library to your project. This library provides the necessary components and functionalities to interact with Excel.
Here are the steps to add the Microsoft Excel 12.0 Object Library to your VB.NET project:
- Open your VB.NET project in Visual Studio.
- Go to the "Solution Explorer" window.
- Right-click on the "References" node and select "Add Reference."
- In the "Reference Manager" window, navigate to the "COM" tab.
- Scroll down and locate "Microsoft Excel 12.0 Object Library" or a similar version, depending on the Excel version installed on your system.
- Check the checkbox next to the library and click "OK" to add the reference to your project.
- Once you have added the reference, you can start working with Excel in your VB.NET code.
From the following pictures to show how to add Excel reference library in your project.
1. Create a new project and add a button to the Form.
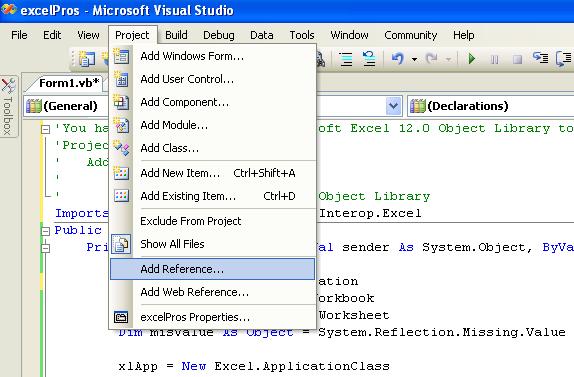
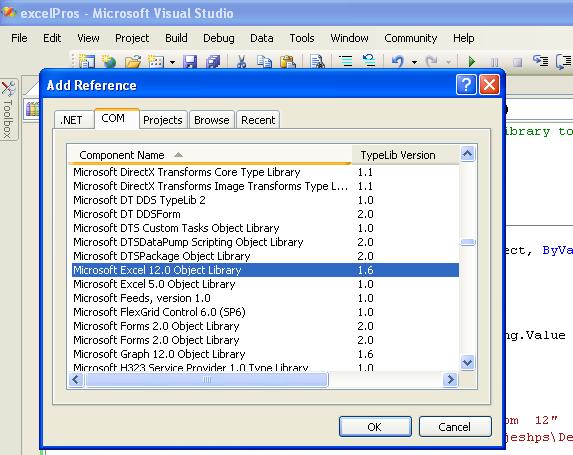
Now you can start coding to open or read from Excel file and edit cells.
Full Source VB.NET In the code , Imports Excel = Microsoft.Office.Interop.Excel - we assign the excel reference to a vatriable Excel.
When you execute this program , the program open the file c:\test1.xlsx and edit the content in the cell B2, it replace the old content to "https://net-informations.com/vb/default.htm" . Before running this program you have to create an excel file name test1.xlsx and add some data in the cell B2.
- How to create an Excel file in VB.NET
- How to read an Excel file using VB.Net
- Add new worksheet in Excel file - VB.Net
- Delete worksheet from an excel file - VB.Net
- How to Format Excel Page in VB.NET
- How to insert a Picture in Excel through programing on VB.NET
- How to insert a background Picture in Excel through VB.NET
- How to create a Chart in Excel in VB.NET
- How to export a Chart in Excel as Picture file from VB.NET
- How to Excel Chart in VB.NET Picture Box
- How to Excel DataBar in VB.NET
- How to Excel Data Validation Input Message
- Read and Import Excel File into DataSet or DataTable in vb.net
- How to insert cell data in an Excel file using OLEDB
- How to update cell data in an Excel file using OLEDB
- How to export from database to excel
- How to export from DataGridView to excel in VB.Net