How to read an Excel file using VB.Net
The following program demonstrates the process of opening an existing Excel spreadsheet in VB.NET, using the COM interop capability provided by the .NET Framework. Additionally, this program provides insights on how to efficiently identify the last used row within the Excel worksheet and how to locate and retrieve named ranges present within the Excel file.
Excel Data Reader using Excel Interop
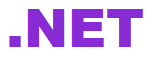
The provided code snippet demonstrates the utilization of the Microsoft Excel 12.0 Object Library in VB.Net to read data from an Excel file. As previously discussed, we have learned the procedure to import the Microsoft Excel 12.0 Object Library into a VB.Net project in the preceding section.
How to add Excel LibraryReading Cells in Excel Using VB.NET
If you want to select a specific cell in Excel sheet, you can code like this.
Using EXCEL Named Ranges in VB.NET
If you want to select multiple cell value from Excel sheet, you can code like this.
How to get the range of occupied cells in excel sheet
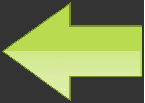
To read all the utilized rows of an Excel file, it is necessary to determine the number of cells that have been used within the file. To accomplish this, we can employ the "UsedRange" property of the xlWorkSheet object. The UsedRange property encompasses all cells that have been utilized, providing us with the last cell within the used area of the worksheet.
When you execute this source code the program read all content from Excel file.
- How to create an Excel file in VB.NET
- How to open or read an existing Excel file in VB.NET
- Add new worksheet in Excel file - VB.Net
- Delete worksheet from an excel file - VB.Net
- How to Format Excel Page in VB.NET
- How to insert a Picture in Excel through programing on VB.NET
- How to insert a background Picture in Excel through VB.NET
- How to create a Chart in Excel in VB.NET
- How to export a Chart in Excel as Picture file from VB.NET
- How to Excel Chart in VB.NET Picture Box
- How to Excel DataBar in VB.NET
- How to Excel Data Validation Input Message
- Read and Import Excel File into DataSet or DataTable in vb.net
- How to insert cell data in an Excel file using OLEDB
- How to update cell data in an Excel file using OLEDB
- How to export from database to excel
- How to export from DataGridView to excel in VB.Net