How to create PDF files in vb.net
The Portable Document Format (PDF) serves as a comprehensive file format that accurately captures and preserves the essential characteristics of a printed document, rendering it readable, writable, printable, and easily shareable. Each PDF file encompasses a comprehensive description of a fixed-layout flat document, encompassing the text, fonts, graphics, and all other necessary elements required for seamless viewing.
PDFsharp library
Within the scope of VB.NET development, creating PDF files programmatically becomes a seamless task. Through the employment of the PDFsharp library, an exceptional Open Source solution, developers can effortlessly generate PDF documents from within their VB.NET applications. This powerful library provides a convenient and efficient means to create PDF files directly from VB.NET applications, eliminating any complexities or hurdles.
You can freely download the Assemblies version from the following link: Download PDFsharp Assemblies
The following steps will guide you how to create a pdf file programmatically
1. Download the Assemblies from the above mentioned url.
2. Extract the .zip file to your desired location (filename :PDFsharp-MigraDocFoundation-Assemblies-1_31.zip)
3. Create a New VB.Net Project
4. Add pdfsharp Assemblies in VB.Net Project
5. In Solution Explorer, right-click the project node and click Add Reference. In this project we are using GDI+ libraries.
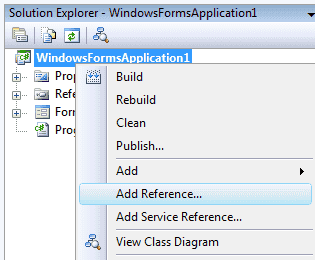
6. In the Add Reference dialog box, select the BROWSE tab and select the Assebly file location (step 2).
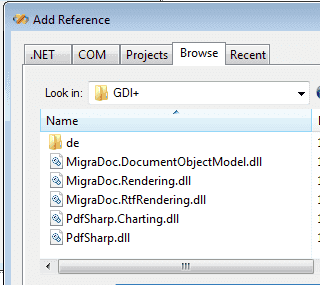
7. Select all files and click OK
After you add the reference files to your VB.Net project , solution explorer look like the following picture.
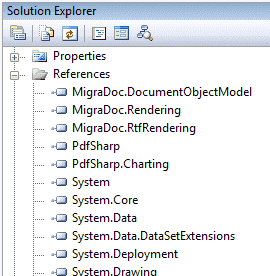
Now the project is ready to start coding
First step you should Imports the necessary namespaces.
Create a PDF document Object
Next step is to create a an Empty page.
Then create an XGraphics Object
Also create the Font object from XFont
After that you can add content to the pdf file
XStringFormats.Center will place the your content to the center of the PDF page.
Save the document as .pdf at your desired location
After save the file , you can double click and open the pdf file. Then you can see the following content in your pdf file.

Drag a Button on the Form and copy and paste the following code in the button1_Click event.
Full Source VB.NETConclusion
PDF serves as a versatile file format that encapsulates all aspects of a printed document, enabling seamless reading, writing, printing, and sharing. Within the field of VB.NET development, the PDFsharp library serves as an invaluable resource, empowering developers to effortlessly create PDF files from their applications. Through the library's comprehensive functionality, developers can seamlessly generate high-quality PDF documents, tailored to their precise specifications, ultimately enhancing the overall efficacy and professionalism of their VB.NET applications.