How to create Dynamic Controls in VB.NET ?
How to create Control Arrays in VB .NET ?
In VB6, Control Arrays is a powerful feature that allows you to group multiple controls of the same type under a single name and assign them individual index values. This enables you to perform operations on the controls dynamically using loops or other iterative processes.
By assigning the same name to a group of controls and differentiating them with unique index values, you create a Control Array. This array-like structure allows you to access and manipulate the controls programmatically in a more efficient and organized manner.
One of the significant advantages of Control Arrays is the ability to loop through the array and set values for each control dynamically. For example, you can iterate through the array using a loop construct such as For...Next or For Each, and assign values to properties or perform other operations on each control within the array.
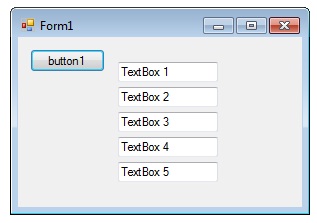
By utilizing this looping mechanism, you can eliminate the need to manually write code for each individual control. Instead, you can write a single block of code that operates on the controls collectively, making your code more concise and maintainable.
How to create Dynamic Controls in VB.NET ?
In the transition from VB6 to VB.NET, one notable feature that is no longer supported is the automated creation of control arrays by duplicating an existing control multiple times into an array. This convenient functionality allowed developers to easily manage and manipulate groups of controls with identical behaviors and properties.
However, in VB.NET, this automated creation of control arrays through simple copying is no longer available. The design paradigm and underlying architecture of VB.NET necessitated a shift away from this approach. Instead, VB.NET emphasizes object-oriented programming principles and encourages the use of more flexible and scalable techniques for control management.
The good news is that, despite the absence of direct support for control arrays, VB.NET provides alternative methods to achieve similar functionality. Developers can still accomplish similar tasks by employing dynamic control creation and management techniques.
The following program shows how to create a dynamic TextBox control in VB.NET and setting the properties dynamically for each TextBox control. Drag a Button control in the form and copy and paste the following source code . Here each Button click the program create a new TextBox control dyanmically.
Full Source VB.NETRather than relying on the automated creation of control arrays, developers can implement efficient algorithms and data structures to manage groups of controls programmatically. This might involve using collections, lists, or other suitable data structures to store and manage the dynamically created controls.
While the transition from VB6 to VB.NET may require some adjustments in control management techniques, the versatility and power of VB.NET offer ample opportunities to accomplish similar tasks through alternative means. By embracing the object-oriented nature of VB.NET and exploring dynamic control creation and management, developers can overcome the absence of control arrays and design effective solutions that meet their specific requirements.
Conclusion
Although control arrays are not directly supported in VB.NET, by dynamically creating controls and managing them at runtime, you can achieve similar behavior and accomplish your desired functionality.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How do i keep a form on top of others
- Timer Control - VB.Net