KeyPress event in VB.NET
In Windows Forms, keyboard input is managed by the framework through the generation of keyboard events in response to Windows messages. When a user interacts with the keyboard, Windows Forms processes the input and raises specific keyboard events, allowing developers to handle and respond to the user's actions.
To effectively process keyboard input in Windows Forms applications, developers typically handle the keyboard events provided by the framework. The two primary keyboard events used for detecting physical key presses are the KeyDown and KeyUp events.
The order in which these key events occur is as follows:
- KeyDown: Triggered when a physical key is pressed down.
- KeyPress: Raised for character keys after the KeyDown event, providing character input.
- KeyUp: Raised when a physical key is released after being pressed down.
KeyDown event
The KeyDown event is triggered when a physical key is pressed down on the keyboard. It provides developers with the opportunity to capture and respond to the key press in real-time. By handling this event, you can perform actions or execute code based on the specific key that was pressed.
KeyPress event
The KeyPress event occurs after the KeyDown event and is raised for character keys. This event provides a way to capture and handle character input from the keyboard. It is useful for scenarios where you need to process specific characters or perform text-related operations.
KeyUp event
The KeyUp event is raised when a physical key is released after being pressed down. It gives developers the ability to respond to key releases and perform actions accordingly. This event is often used in conjunction with the KeyDown event to track the complete lifecycle of a key press, from pressing down to releasing the key.
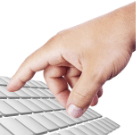
What it captures:
- Printable characters (alphabets, numbers, symbols)
- Space key
- Backspace key
What it doesn't capture:
- Non-printable characters (function keys, arrow keys, Ctrl, Shift, Alt)
- Combinations of keys (these are handled by KeyDown and KeyUp events)
How to use it:
- Set the Focus: The control (like a text box) needs to have focus for the KeyPress event to work.
- Event Handler: In the properties of the control, you can double-click on the KeyPress event to create an event handler function.
- Accessing Key Information: Inside the event handler function, you'll have access to the KeyEventArgs object (represented by e in most examples). This object provides properties like: e.KeyChar: This holds the character that was pressed (if applicable).
How to detect when the Enter Key Pressed in VB.NET
The following VB.NET code behind creates the KeyDown event handler. If the key that is pressed is the Enter key, a MessegeBox will displayed .
How to get TextBox1_KeyDown event in your VB.Net source file ?
Select your VB.Net source code view in Visual Studio and select TextBox1 from the top-left Combobox and select KeyDown from top-right combobox , then you will get keydown event sub-routine in your source code editor.
Difference between the KeyDown Event, KeyPress Event and KeyUp Event in VB.Net
VB.Net KeyDown Event : This event raised as soon as the person presses a key on the keyboard, it repeats while the user hold the key depressed.
VB.Net KeyPress Event : This event is raised for character keys while the key is pressed and then released by the use . This event is not raised by noncharacter keys, unlike KeyDown and KeyUp, which are also raised for vb.net noncharacter keys
VB.Net KeyUp Event : This event is raised after the person releases a key on the keyboard.
VB.Net KeyPress Event
VB.Net KeyDown Event
How to Detecting arrow keys in VB.Net
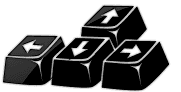
In order to capture keystrokes in a VB.Net Forms control, you must derive a new class that is based on the class of the control that you want, and you override the ProcessCmdKey().
VB.Net KeyUp Event
The following VB.NET source code shows how to capture Enter KeyUp event from a TextBox Control.
Full Source VB.NETHere are some additional points to consider:
- KeyPreview: To capture keypress events even when other controls have focus, set the KeyPreview property of the form to True.
- Comparison: KeyPress differs from KeyDown and KeyUp events. KeyDown fires when any key is pressed, KeyUp fires when a key is released, and KeyPress focuses on the character generated by the keypress.
Conclusion
Handling these key events in the appropriate order, you can effectively process and respond to keyboard input within your Windows Forms application. This allows for dynamic and interactive user experiences, where user actions can be captured and appropriate actions can be performed based on the pressed keys.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net