OpenFile Dialog Box in VB.Net
The OpenFileDialog component in VB.Net provides a convenient way to display a dialog box that allows users to select a file for opening within an application. By utilizing this component, you can incorporate file selection functionality seamlessly into your VB.Net application.
The OpenFileDialog dialog box offers users the flexibility to navigate through their file system and choose the desired file to open. The dialog box provides an intuitive and familiar interface for browsing folders and selecting files.
FileName property
Before displaying the OpenFileDialog, you have the option to set the FileName property programmatically. By assigning a value to this property prior to showing the dialog box, you can instruct the dialog box to initially display the specified filename. This feature is particularly useful when you want to prepopulate the dialog box with a specific file or provide a default filename to the user.
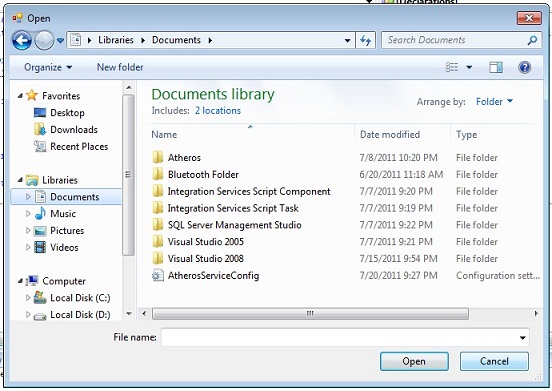
The FileName property can be set prior to showing the dialog box. This causes the dialog box to initially display the given filename. In most cases, your applications should set the InitialDirectory, Filter, and FilterIndex properties prior to calling ShowDialog.
However, in most cases, it is recommended to set a few essential properties of the OpenFileDialog component before invoking the ShowDialog method. These properties include:
- InitialDirectory: This property allows you to specify the initial directory that the OpenFileDialog will display when opened. It can be set to a specific folder path or to a special value such as Desktop or MyDocuments to provide a starting point for file navigation.
- Filter: The Filter property enables you to define the types or extensions of files that the OpenFileDialog will display to the user. By setting this property, you can restrict the visible files to specific formats or categories, providing users with a filtered view based on their needs.
- FilterIndex: This property determines the index of the default filter within the Filter property. It specifies which filter option will be selected by default when the OpenFileDialog is opened. By setting this property, you can streamline the file selection process by preselecting a commonly used file type.
The following VB.Net program invites an OpenFile Dialog Box and retrieve the selected filename to a string.
Full Source VB.NETConclusion
The OpenFileDialog component in VB.Net simplifies the process of file selection by displaying a dialog box that allows users to choose a file to open. By configuring properties such as FileName, InitialDirectory, Filter, and FilterIndex, you can tailor the OpenFileDialog to suit your application's needs and ensure a smooth and efficient file selection process for your users.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net