Checked ListBox Control in VB.Net
The CheckedListBox control in VB.Net provides all the features and functionality of a standard ListBox control, with the added capability of displaying a check mark alongside each item in the list. This additional functionality allows users to select multiple items from the list by checking the corresponding checkboxes.
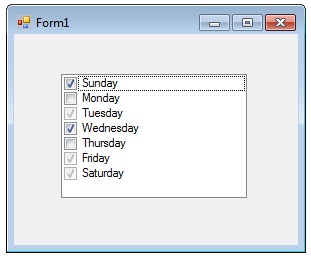
The CheckedListBox control enables users to toggle the selection state of each item independently by clicking on the associated checkbox. The state of each checkbox (checked or unchecked) can be programmatically accessed and manipulated to perform actions based on the user's selections.
AddRange method
To dynamically add objects to a CheckedListBox at runtime in VB.Net, you can utilize the AddRange method. This method allows you to assign an array of object references, which will then be displayed as items in the CheckedListBox.
By using the AddRange method, you can efficiently add multiple objects to the CheckedListBox in a single operation, rather than adding them one by one. The objects can be of any type, and the CheckedListBox will display the default string representation of each object.
Upon adding the objects, the CheckedListBox will automatically generate the default string value for each object. This string representation is typically obtained by calling the ToString method on each object. It's important to ensure that the objects in the array have a meaningful ToString implementation to display relevant information in the CheckedListBox.
You can add individual items to the list with the Add method. The CheckedListBox object supports three states through the CheckState enumeration: Checked, Indeterminate, and Unchecked.
Conclusion
By using the CheckedListBox control, you can provide a versatile and user-friendly interface for managing multiple selections within your VB.Net application.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net