ComboBox Control | VB.Net
In the VB.Net development environment, a wide range of controls can be found within the Toolbox. These controls serve as essential building blocks, allowing developers to effortlessly create objects on a form through a straightforward series of mouse clicks and dragging motions.
Among the multitude of controls available, the ComboBox control holds significant importance. The ComboBox control provides a versatile user interface element that allows users to choose from a selection of options. Users can either input a value directly into the text field or click a button to reveal a drop-down list containing predefined choices.
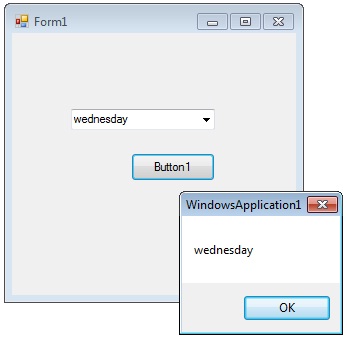
In addition to its display and selection functionality, the ComboBox control offers additional features that streamline the process of adding items to the control. This efficiency allows developers to conveniently populate the ComboBox with options, enhancing the user experience by presenting a comprehensive range of choices.
Add item to combobox
How to set the selected item in a comboBox
You can set which item should shown while it displaying in the form for first time.
ComboBox SelectedItem
How to retrieve value from ComboBox
If you want to retrieve the displayed item to a string variable , you can code like this
How to remove an item from ComboBox in VB.Net
You can remove items from a combobox in two ways. You can remove item at a the specified index or giving a specified item by name.
The above code will remove the second item from the combobox.
The above code will remove the item "Friday" from the combobox.
DropDownStyle
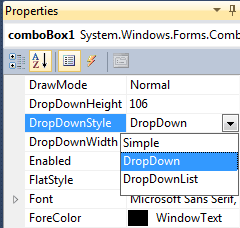
The DropDownStyle property specifies whether the list is always displayed or whether the list is displayed in a drop down. The DropDownStyle property also specifies whether the text portion can be edited.
ComboBox DataSource Property
Programmatically Binding DataSource to ComboBox
You can populate a combo box with a DataSet in a simple way..
Consider an sql query string like...."select au_id,au_lname from authors";
Make a datasource in your program and bind it like the following...
Combobox SelectedIndexChanged event
The SelectedIndexChanged event of a ComboBox is triggered whenever there is a modification in the selected item within the ComboBox control. This event provides an opportunity to execute specific actions or code when the selection undergoes a change. By utilizing the SelectedIndexChanged event, you can implement custom behaviors and logic that respond dynamically to the user's selection alterations.
To gain insight into the process of setting values within the SelectedIndexChanged event of a ComboBox, follow these steps:
Output
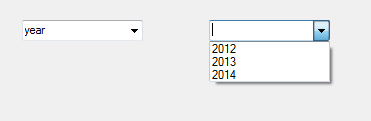
ComboBox Default Value
How to set a default value for a Combo Box
You can set combobox default value in VB.Net by using SelectedIndex property
Above code set 6th item as combobox default value
ComboBox readonly
How to make a combobox read only
There are two approaches to make a ComboBox read-only, preventing the user from directly typing into it while still allowing them to select from the provided values.
The first method involves modifying the DropDownStyle property of the ComboBox. By default, the DropDownStyle is set to "DropDown," which allows the user to both select from the list and input their own values. However, changing the DropDownStyle property to "DropDownList" makes the ComboBox read-only. In this mode, the user can only choose from the predefined values without the ability to enter custom input.
The second method involves disabling the ComboBox by setting the Enabled property to "False." This completely renders the ComboBox as read-only, preventing any interaction or selection by the user.
Both methods effectively achieve a read-only state for the ComboBox, but they differ in the level of user interaction permitted. The first method limits input capability while still enabling selection from the list, while the second method completely disables any user interaction with the ComboBox.
Choose the method that best suits your requirements and implement it accordingly to achieve the desired read-only behavior for your ComboBox in VB.Net.
ComboBox Example
The following VB.Net source code add seven days in a week to a combo box while load event of a Windows Form and display the fourth item in the combobox.
Full Source VB.NETConclusion
The ComboBox control, with its diverse set of features and capabilities, serves as a powerful tool for gathering user input and providing a seamless selection experience within VB.Net applications.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net