ListBox Control in VB.Net
VB.Net offers various mechanisms for gathering input within a program, and one of the options is the ListBox control from Windows Forms. The ListBox control serves as a user interface element that presents a list of choices from which the user can select.
When utilizing the ListBox control, you can populate it with a set of options that are displayed to the user. The user can then make a selection from the available choices by clicking on an item in the list. This interactive functionality allows for the collection of user input in a structured and controlled manner.
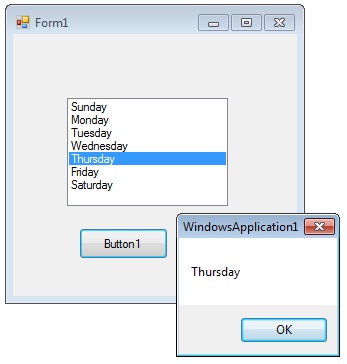
The ListBox control is designed to accommodate scenarios where the user is presented with multiple options and needs to make a single selection. It provides an intuitive and efficient way to present choices and gather input within a VB.Net program's user interface.
When working with a ListBox in VB.Net, you have the flexibility to add items to the list using the Add or Insert methods. These methods provide convenient ways to populate the ListBox with new items according to your specific requirements.
The Add method is used to append new items to the end of an unsorted ListBox. By invoking the Add method, you can seamlessly append new items to the existing list, ensuring they are displayed at the bottom of the list.
On the other hand, the Insert method enables you to specify the desired position at which to insert a new item in the ListBox. This allows for precise control over the order and placement of items within the list. By specifying the index at which the item should be inserted, you can ensure it appears exactly where you intend it to be in the list.
Both the Add and Insert methods serve as effective means of adding items to a ListBox. The choice between them depends on whether you want to append items to the end of the list or insert them at a specific position within the existing items.
The SelectionMode property determines how many items in the list can be selected at a time. A ListBox control can provide single or multiple selections using the SelectionMode property .
If you want to retrieve a single selected item to a variable , you can code like this.
If you change the selection mode property to multiple select , then you will retrieve a collection of items from ListBox1.SelectedItems property.
The following VB.Net program initially fill seven days in a week while in the form load event and set the selection mode property to MultiSimple. At the Button click event it will display the selected items.
Full Source VB.NETConclusion
By incorporating the ListBox control in your program, you can enhance the user experience and facilitate the selection of choices from a list of options, ensuring a smooth and interactive input process.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net