ListView Control in VB.Net
The ListView control in VB.Net provides a versatile and visually appealing way to display a collection of items. The ListView control offers five different views to present the items: LargeIcon, Details, SmallIcon, List, and Tile. Each view has its own distinct layout and style, allowing developers to choose the most suitable presentation for their data.
What You Can Do with the ListView Control
The ListView control offers a versatile way to display and manage lists of items. You can populate it with text, icons, or a combination of both. It allows users to select single or multiple items, making it suitable for tasks like choosing files, browsing options, or displaying search results. You can even add checkboxes to your ListView for creating selectable lists with on/off options.
Add Columns in VB.Net ListView
To add columns to a ListView, the Columns.Add() method is utilized. This method enables you to define the columns and their respective properties within the ListView control. The Columns.Add() method accepts two arguments: the column heading and the column width.
In the above code, "ProductName" is column heading and 100 is column width.
By specifying the column heading as the first argument, you provide a descriptive label for the column, indicating the type of information or attribute being displayed in that column. This helps users understand the purpose or content of the column.
The second argument of the Columns.Add() method is the column width. This determines the width of the column within the ListView control. You can specify the width in pixels or as a relative value, depending on your specific requirements and layout preferences.
Add Item in VB.Net Listview
You can add items in listbox using ListViewItem which represents an item in a ListView control.
Get selected item from VB.Net ListView
Above code will return the itme from first column of first row.
Sorting VB.Net Listview Items
If the Sorted property of Listview is set to true, then the ListView items are sorted. The following code sorts the ListView items:
Add Checkbox in Listview
You can add checkbox in VB.Net Listview columns.
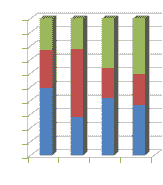
ListView provides a large number of properties that provide flexibility in appearance and behavior. The View property allows you to change the way in which items are displayed. and the SelectionMode property determines how many items in the list can be selected at a time.
The following Vb.Net program first set its view property as Details and GridLines property as true and FullRowSelect as true.
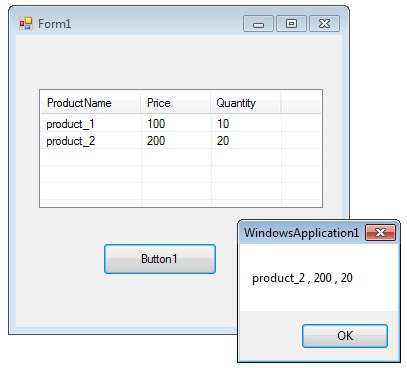
Finally in the button click event, it will display the selected row values in a message box.
Full Source VB.NETConclusion
By utilizing the Columns.Add() method, you can enhance the ListView control by incorporating columns that organize and present your data in a structured and easily readable manner, improving the overall user experience and data visualization within your VB.Net application.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net