ScrollBars Control in VB.Net
In VB.Net, the ScrollBar control is used to provide users with a graphical interface element for scrolling through content that exceeds the visible area of a container, such as a form or a panel. The ScrollBar control enables users to navigate vertically or horizontally within the container to access hidden or off-screen content.
Key aspects of the ScrollBar control
The ScrollBar control comes with several properties, events, and methods that allow for customization and interactivity. Let's explore some key aspects of the ScrollBar control:
- Orientation: The ScrollBar control can be oriented vertically or horizontally, depending on the layout and scrolling requirements of the container.
- Minimum and Maximum values: The Minimum and Maximum properties define the range of values that the ScrollBar represents. These values determine the scrolling boundaries and are typically set to reflect the size or extent of the content being scrolled.
- Value property: The Value property specifies the current position of the ScrollBar within the defined range. By manipulating this property, you can programmatically scroll to a specific location.
- SmallChange and LargeChange properties: These properties determine the amount of content to be scrolled when the user clicks the small increment or large increment areas of the ScrollBar, respectively.
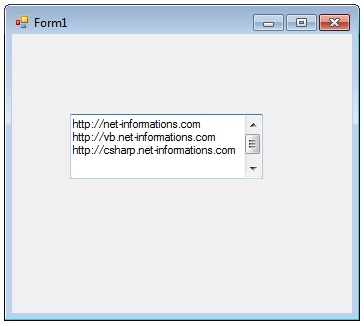
To illustrate the usage of the ScrollBar control, consider a scenario where you have a large text box containing lengthy content. You can place a vertical ScrollBar control alongside the text box, enabling users to scroll through the text vertically. Here's an example:
In this example, the ScrollBar's ValueChanged event is handled to update the position of the TextBox based on the current value of the ScrollBar. As the user interacts with the ScrollBar, the TextBox's location is adjusted accordingly, creating the scrolling effect.
Conclusion
By using the ScrollBar control, you can enable users to navigate through content that extends beyond the visible area, enhancing the user experience and providing a means to access hidden information or large data sets within your VB.Net application.
- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net TextBox Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net