TextBox Control | VB.Net
In VB.Net, a variety of mechanisms are available to facilitate input gathering within a program. One such mechanism is the TextBox control, which serves the purpose of displaying or accepting a single line of text as input. Within the field of VB.Net programming, developers extensively utilize the TextBox control to enable users to view or enter substantial amounts of text.
A TextBox control is employed as an object to display textual content on a form, allowing users to both view and input data during the execution of a VB.Net program. Through the TextBox control, users can interactively type in data or even paste information from the clipboard, providing a flexible and efficient means of data entry.
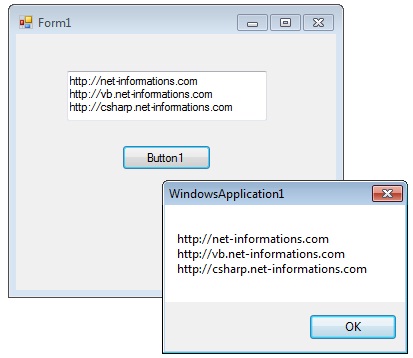
The versatility of the TextBox control, in terms of displaying and gathering text, makes it a fundamental element in user interface design for VB.Net applications. By using this control, developers can empower users to interact with the program through the input and manipulation of textual information.
For displaying a text in a TextBox control , you can code like this.
You can also collect the input value from a TextBox control to a variable like this way.
VB.Net TextBox Properties
The configuration of TextBox properties can be accomplished through two primary methods: the Property window and programmatic manipulation. The Property window, usually found within the solution explorer, provides a convenient graphical interface for modifying control properties. To access the Property window, you can either press the F4 key or right-click on a TextBox control and select the "Properties" menu item.
By opening the Properties window, you gain access to a comprehensive list of configurable properties for the TextBox control. Through this interface, you can easily modify various aspects such as text content, appearance, behavior, and interaction with the user.
Alternatively, you can also programmatically manipulate TextBox properties within your code. This allows for dynamic control over the TextBox behavior and appearance based on specific conditions and user interactions. By accessing the TextBox control's properties directly in your code, you can customize and control its behavior in a more flexible and tailored manner.
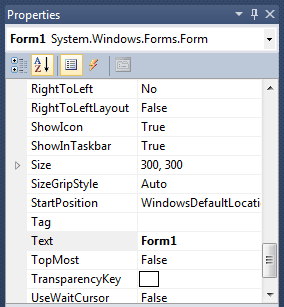
The below code set a textbox width as 150 and height as 25 through source code.
Background Color and Foreground Color
You can set Textbox background color and foreground color through property window, also you can set it programmatically.
TextBox BorderStyle
You can set 3 different types of border style for textbox in vb.net, they are None, FixedSingle and fixed3d.
VB.Net TextBox Events
TextBox Keydown event
Keydown event occurs when a key is pressed while the control has focus.
e.g.
TextChanged Event
TextChanged Event is raised if the Text property is changed by either through program modification or user input.
e.g.
VB.Net Textbox Maximum Length
Maximum Length property sets the maximum number of characters or words the user can input into the text box control. That means you can limit the user input by this property.
How to ReadOnly Textbox
when a program wants to prevent a user from changing the text that appears in a text box, the program can set the controls Readonly property is to True.
Multiline TextBox in vb.net
By default TextBox accept single line of characters , If you need to enter more than one line in a TextBox control, you should change the Multiline property is to True.
Textbox passowrd character
Sometimes you want a textbox to receive password from the user. In order to keep the password confidential, you can set the PasswordChar property of a textbox to a specific character.
The above code set the PasswordChar to * , so when the user enter password then it display only * instead of typed characters.
How to Newline in TextBox
You can add new line in a textbox using two ways.
or
How to retrieve integer values from textbox ?
VB.Net String to Integer conversion
Parse method Converts the string representation of a number to its integer equivalent.
VB.Net String to Double conversion
From the following VB.Net source code you can see some important property settings to a TextBox control.
Full Source VB.NET- Visual Studio IDE
- How to Create a VB.Net Windows Forms Application
- Label Control | VB.Net
- VB.Net Button Control
- VB.Net ComboBox Control
- VB.Net ListBox Control
- VB.Net Checked ListBox Control
- VB.Net RadioButton Control
- VB.Net CheckBox Control
- VB.Net PictureBox Control
- VB.Net ProgressBar Control
- VB.Net ScrollBars Control
- VB.Net DateTimePicker Control
- VB.Net Treeview Control
- VB.Net ListView Control
- VB.Net Menu Control
- VB.Net MDI Form
- VB.Net Color Dialog Box
- VB.Net Font Dialog Box
- VB.Net OpenFile Dialog Box
- VB.Net Print Dialog Box
- KeyPress event in VB.NET
- How to create Dynamic Controls in VB.NET ?
- How do i keep a form on top of others
- Timer Control - VB.Net