How to use Enum in vb.net
When you are in a situation to have a number of constants that are logically related to each other, you can define them together these constants in an enumerator list. An enumerated type is declared using the enum keyword.
An enumeration comprises a designated name, an underlying data type, and a collection of members, with each member representing a constant value. This construct proves particularly valuable when working with a predefined set of values that hold functional significance and remain fixed throughout the program's execution. By defining an enumeration, developers can establish a clear and meaningful representation of these constant values, enhancing code readability and maintainability. The underlying data type of the enumeration ensures type safety and facilitates seamless integration with other components of the program.
Syntax: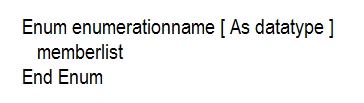
A simple Enum Excercise
Initializing Members
By default, each element in the enum is assigned an underlying type of int. If you do not explicitly specify an initializer for a member, VB.Net automatically initializes it to zero. To illustrate this behavior, let's consider the previous example and attempt to convert the enum values to integers. The result would be as follows:
Output is : Temperature value is..1
Retrieve and check the Enum value
When declaring an enum, if you assign a specific value to the first member, the subsequent members will be automatically assigned values greater by one than that of the immediately preceding member. You can observe this behavior in the following program:
Output is : Temperature value is..4
You can specify another integral numeric type by using a colon. The following Enum declare as byte, you can verify the underlying numeric values by casting to the underlying type.
You can retrieve the value like the following:
How to get int value from enum
Enum.Parse in VB.Net
The Enum.Parse() method in VB.Net allows you to convert a string representation or integer value of one or more enumerated constants into an equivalent Enum object. This method enables you to dynamically convert a string or integer value into its corresponding Enum value during runtime. By specifying the appropriate Enum type and the string or integer value, Enum.Parse() performs the conversion and returns an Enum object that represents the specified value. This functionality is particularly useful when working with user input or when you need to convert values between different representations within your code.
String convert to Enum
Output is : green
How can an int value cast to enum
Following is the easiest method to cast an int to enum.
MyEnum myenum = (MyEnum)intvalue;
Ex:
Converts int to enum values
Method 2:
MyEnum myenum = (MyEnum)Enum.ToObject(typeof(MyEnum) , intvalue);
You can check if it's in range using Enum.IsDefined
How to Loop through all enum values in VB.Net
The GetValues() method in VB.Net allows you to retrieve an array that contains a value for each member of the enum Type. This method provides a convenient way to access and iterate over all the possible values of an enum. The array returned by GetValues() includes a value for each distinct member of the enum, even if multiple members have the same value. This means that if there are duplicate values within the enum, the resulting array will include duplicate values as well. This behavior ensures that all possible enum values are accounted for and accessible through the returned array.
Iterating through an enum in vb.net
The following example will show how do enumerate an enum .
Enum in Select Case
The following program shows how to use Enum in Select....Case statement.
Full Source VB.NETConclusion
An Enum can be used to define a set of related constants, providing a structured and readable way to work with fixed values, such as days of the week or status codes, by assigning a name and underlying value to each constant.