How to use FOR NEXT loop in vb.net
In programming, when you encounter a scenario where a specific task needs to be repeated multiple times or iterated until a certain condition is met, loop statements come to the rescue. These loop statements provide a convenient way to achieve the desired results by executing a block of code repeatedly.
FOR NEXT loop
One such loop statement is the "FOR NEXT" loop, which proves particularly useful when you know in advance the exact number of times you want the loop to iterate. This type of loop is commonly employed when iterating over arrays or when a predetermined number of iterations is required for a specific application.
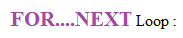
The "FOR NEXT" loop executes the loop body, which encompasses the source code within the For...Next code block, a fixed number of times. This fixed number, known as the loop count or iteration count, is determined by specifying the starting value, ending value, and optional step value in the loop declaration.
By defining the loop count and establishing the iteration parameters, you can ensure that the loop iterates precisely the desired number of times. During each iteration, the loop variable (typically defined in the loop declaration) is automatically incremented or decremented according to the specified step value, allowing for precise control over the loop flow.
- var : The counter for the loop to repeat the steps.
- starValue : The starting value assign to counter variable.
- endValue : When the counter variable reach end value the Loop will stop.
- loopBody : The source code between loop body.
Lets take a simple real time example , If you want to show a messagebox 5 times and each time you want to see how many times the message box shows.
- Line 1: Loop starts value from 1.
- Line 2: Loop will end when it reach 5.
- Line 3: Assign the starting value to var and inform to stop when the var reach endVal.
- Line 4: Execute the loop body.
- Line 5: Taking next step , if the counter not reach the endVal.
When you execute this program , It will show messagebox five time and each time it shows the counter value.
If you want to Exit from FOR NEXT Loop even before completing the loop Visual Basic.NET provides a keyword Exit to use within the loop body.
The "FOR NEXT" loop is a powerful tool for managing repetitive tasks that require a known number of iterations. Whether you need to process elements in an array, perform calculations, or execute a set of instructions a fixed number of times, the "FOR NEXT" loop simplifies the implementation and streamlines your code.
Optimize the code
By explore the capabilities of the "FOR NEXT" loop, programmers can effectively optimize their code and achieve efficient execution, as they can precisely define the number of iterations required. This control over the loop count empowers developers to create robust and reliable applications, ensuring that specific tasks are repeated the desired number of times.
Full Source VB.NETConclusion
Loop statements, such as the "FOR NEXT" loop, offer a powerful mechanism for repeating tasks or iterating until a specific condition is met. With the ability to specify the iteration count in advance, the "FOR NEXT" loop provides developers with fine-grained control over their code's repetitive execution, enhancing both the efficiency and clarity of their programs.