How to use IF ELSE in VB.NET
The conditional statement "IF...ELSE" is a fundamental construct in programming that allows for the examination of provided conditions and facilitates decision-making based on those conditions. This statement serves as a crucial tool for controlling the flow of a program based on specific criteria.
When using the IF...ELSE statement, developers can evaluate various conditions by employing comparison operators, such as "equals to" (==), "greater than" (>), "less than" (<), "not equal to" (!=), and others. These operators enable the comparison of values or variables to determine if they meet certain criteria or relationships.
Additionally, logical operators, such as "AND," "OR," and "NOT," can be used within the conditional statement to combine multiple conditions or negate a condition. This allows for more complex and comprehensive evaluations, where multiple conditions need to be satisfied or specific conditions need to be excluded.
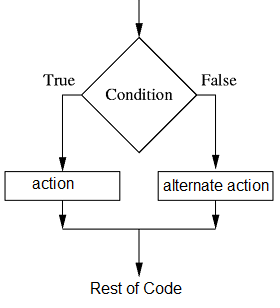
By utilizing the IF...ELSE statement in combination with comparison and logical operators, developers can effectively analyze data or variables and make informed decisions within their programs. This decision-making process enables the program to dynamically respond to different scenarios or user inputs, resulting in more flexible and adaptive behavior.
The ability to examine conditions and make decisions based on them is a fundamental aspect of programming logic. The IF...ELSE statement, together with comparison and logical operators, empowers developers to incorporate conditional branching and logic into their code, providing a mechanism for implementing different paths or actions based on the evaluation of conditions.
If the contition is TRUE then the control goes to between IF and Else block , that is the program will execute the code between IF and ELSE statements.
If the contition is FLASE then the control goes to between ELSE and END IF block , that is the program will execute the code between ELSE and END IF statements.
If you want o check more than one condition at the same time , you can use ElseIf .
Just take a real-time example - When we want to analyze a mark lists we have to apply some conditions for grading students depends on the marks.
Following are the garding rule of the mark list:
1) If the marks is greater than 80 then the student get higher first class
2) If the marks less than 80 and greater than 60 then the student get first class
3) If the marks less than 60 and greater than 40 then the student get second class
4) The last condition is , if the marks less than 40 then the student fail.
Now here implementing these conditions in a VB.NET program.
- Line 1 : Checking the total marks greaterthan or equal to 80.
- Line 2 : If total marks greater than 80 show message - "Got Higher First Class ".
- Line 3 : Checking the total marks greaterthan or equal to 60.
- Line 4 : If total marks greater than 60 show message - "Got First Class ".
- Line 5 : Checking the total marks greaterthan or equal to 40.
- Line 6 : If total marks greater than 40 show message - "Just pass only".
- Line 7 : If those three conditions failed program go to the next coding block.
- Line 8 : If all fail shows message "Failed".
- Line 9 : Ending the condition block.
In this example the total marks is 59 , when you execute this program you will get in messagebox "Just Pass Only"
If you want to check a condition within condition you can use nested if statements.
Also you can write IF ELSE Statements in a single line.
Conclusion
Skillfully utilizing conditional statements and operators, developers can enhance the functionality and interactivity of their programs, creating intelligent systems that respond intelligently to varying inputs or situations. This level of control ensures that programs can make appropriate decisions and take specific actions based on the conditions they encounter during execution.