Remote Client Application in VB.Net
The process of calling methods on a Remote Object in VB.NET is straightforward and follows a simple pattern. When a client application invokes a method on a Remote Object, the .NET Remoting system intercepts the call, routes it to the appropriate remote object, and returns the results back to the client.
Remote Object
To enable the client application to interact with the Remote Object, it is necessary to register the Remote Type. This registration process ensures that the client application is aware of the Remote Object's structure and functionality, allowing it to create instances and invoke methods on the Remote Object.
In the client application written in VB.NET, the first step involves creating an instance of the RemoteTime object, which represents the Remote Object. Once the instance is created, the client application can proceed to call the desired method, such as getTime(), to retrieve the relevant information or perform the desired operation.
Full Source VB.NETCopy and paste the above VB.Net source code into a file and save it as Client.vb.
It is worth noting that the client application relies on a configuration file, Client.exe.config, to provide communication information to the Remoting Framework. This configuration file contains crucial details, such as network settings and endpoint configurations, enabling the Remoting Framework to establish the necessary communication channels and facilitate the interaction between the client application and the Remote Object.
We have to create additional configuration file to provide the communication information to the Client Object. The configuration file is a an XML structured file. We can specify the Remote Type , Channel , port for communication etc. through the configuration file .
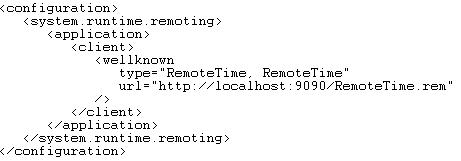
Click here to download Client.exe.config .
Compile the class file Client.vb using the command-line tools that ship with the .NET Framework SDK.
At the command prompt in the directory in which you saved the file, type the following command:
vbc /r:RemoteTime.dll Client.vb
After you compile the Client.vb , you will get a file called Client.exe in the directory which you saved the source file
Full Source VB.NETConclusion
The client application in VB.NET follows a straightforward process to interact with Remote Objects. By registering the Remote Type and creating instances of the Remote Object, the client application can invoke methods and retrieve results. Additionally, the reliance on the configuration file, Client.exe.config, ensures proper communication and configuration settings for the Remoting Framework. These steps collectively enable efficient communication and interaction between the client application and the Remote Object, facilitating the development of robust and distributed systems.