How to VB.NET String.Split()
The Split() method in VB.Net is a powerful tool that allows you to extract substrings from a given string based on a specified separator. It works by identifying the occurrences of the separator within the original string and splitting it into multiple substrings. These extracted substrings are then returned as separate elements within an array.
What it does:
- Takes a string as input.
- Splits the string into substrings based on a separator (delimiter).
- Returns an array of strings, where each element represents a substring between delimiters.
Specifying the Delimiter:
- You can use a single character as a delimiter (e.g., comma, space).
- Alternatively, you can use a string as a delimiter (e.g., a specific word).
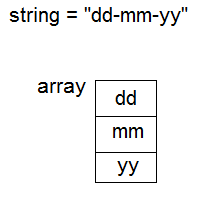
If your String contains "dd-mm-yy", split on the "-" character to get an array of: "dd" "mm" "yy".
If the separator parameter is null or contains no characters, white space characters are assumed to be the delimiters.
Syntax :
- separator - the given delimiter.
- An array of Strings delimited by one or more characters in separator.
Output:
By calling the Split() method on the sentence string and passing the separator as an argument, the method splits the sentence into individual words based on the spaces. The resulting words are stored in an array called "words".
Optional Parameters:- RemoveEmptyEntries: This removes any empty entries (consecutive delimiters) from the resulting array.
- Limit: This limits the number of splits performed. Useful when you only want a certain number of substrings.
- CompareOptions: This controls case-sensitivity during the split operation.
We can then use a loop to iterate over the "words" array and print each word on a separate line. This allows us to access and manipulate the extracted substrings individually.
VB.Net String Split Example
VB.Net String Split by multiple characters delimiter
In addition to splitting a string using a single character delimiter, the String.Split() method in VB.Net also allows us to split a string based on multiple character delimiters. This enables us to extract substrings from the original string by considering a combination of characters as separators.
To split a string using multiple character delimiters, we can provide an array of characters as the separator parameter in the Split() method. The method will then identify any occurrence of these characters in the string and split it accordingly.
Output:
Using Regular Expressions for multiple characters
To split a string by multiple characters delimiter using regular expressions in VB.Net, you can utilize the Regex.Split() method from the System.Text.RegularExpressions namespace. This method allows you to split a string based on a regular expression pattern rather than a specific character or substring.
Output:
Split() a delimited string to a List < String > in VB.Net
You can retrieve the result of a String splt() method to a VB.Net List. The following program convert the String Array to a List.
VB.Net Convert List to String
VB.NetString split White spaces
You can remove all whitespace from a string using the StringSplitOptions.RemoveEmptyEntries option. This option ensures that the return value of the split operation does not include any array elements that contain an empty string.
Full Source VB.NET- If there's no delimiter in the string, Split() returns an array with the entire original string as the sole element.
- Delimiters at the beginning or end of the string result in empty entries in the array (unless RemoveEmptyEntries is used).
Conclusion
By using the String.Split() method with multiple character delimiters, we can easily break down a string into meaningful substrings based on various combinations of characters. This offers flexibility in handling complex string patterns and enhances the versatility of our string manipulation operations.
- How to vb.net String Length()
- How to vb.net String Insert()
- How to vb.net String IndexOf()
- How to vb.net String Format()
- How to vb.net String Equals()
- How to vb.net String CopyTo()
- How to vb.net String Copy()
- How to vb.net String Contains()
- How to vb.net String Compare()
- How to vb.net String Clone()
- How to vb.net String Chars()
- How to vb.net String substring()
- How to vb.net String EndsWith()
- How to vb.net String Concat()
- How to VB.NET String Null