How to open and read XML file in VB.NET
In the previous program, we generated an XML file called "products.xml." Now, we will focus on reading the contents of this XML file and extracting the information enclosed within specific XML tags. There are multiple approaches available for reading an XML file, and the chosen method depends on the specific requirements of the task at hand.
XmlDataDocument class
In this program, we will be reading the XML file in a node-wise manner. To accomplish this, we will utilize the XmlDataDocument class, which provides functionality for parsing and navigating XML data. By employing this class, we can efficiently traverse through the XML file and extract the desired data.
In the program, we target the "<Product>" node and its child nodes to extract the relevant information stored within them. The XmlDataDocument class enables us to access these specific nodes and retrieve the data they contain.
Click here to download the input file : product.xml
How to read XML from a file in VB.Net
Reading Xml with XmlReader in VB.Net
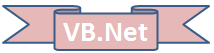
XmlReader is a highly efficient and memory-friendly alternative for reading XML files in VB.NET. It offers a lower-level abstraction over the structure of an XML file, allowing for streamlined processing and reduced memory consumption.
The XmlReader class operates by sequentially parsing the XML string, enabling you to navigate through the XML elements one at a time. This approach ensures a minimal memory footprint since the entire XML document is not loaded into memory at once. Instead, XmlReader reads the XML content in a forward-only manner, making it suitable for scenarios where memory usage is a concern or when dealing with large XML files.
The XmlReader class's design focuses on efficiency and speed, making it an ideal choice when performance optimization is a priority. It is particularly useful when you only need to extract specific data or perform selective operations on the XML file.
VB.Net XML Handling
Full Source : Reading Xml with XmlReader
Reading XML with XmlTextReader in VB.Net
XmlTextReader Class provides forward-only, read-only access to a stream of XML data.
Full Source : Reading Xml with XmlReader
Reading XML with XmlDocument in VB.Net
The XmlDocument class in VB.NET offers a comprehensive approach for reading and manipulating XML files. It provides the capability to load the complete XML content into memory, enabling flexible navigation and querying of the XML document using the XPath technology.
When utilizing XmlDocument, the entire XML content is loaded into memory, allowing for efficient traversal and manipulation of the XML structure. This in-memory representation enables easy navigation and exploration of the XML document by providing methods and properties to access specific elements, attributes, and text nodes.
Click here to download the input file : product.xml
Full Source VB.NETConclusion
XmlReader provides a fast and memory-efficient approach for reading XML files in VB.NET. Its forward-only, sequential processing capability allows for efficient traversal of XML elements and retrieval of their values. When dealing with large XML files or performance-sensitive scenarios, XmlReader offers superior performance and reduced memory consumption compared to higher-level XML parsing approaches.
- How to XML in VB.NET
- How to create an XML file in VB.NET
- How to create an XML file in VB.NET using Dataset
- How to open and read an XML file in VB.NET using Dataset
- How to create an XML file from SQL in VB.NET
- How to search in an XML file
- How to filter data in an XML file
- How to insert data from xml to database
- How to create an Excel file from XML
- How to create an XML file from Excel
- How to xml to DataGridView
- How to create a TreevView from XML
- How to create Crystal Reports from XML
- How to serialization in xml
- How to serialize a .Net Object to XML
- How to de-serialize from an XML file to .Net Object